Batch re-encode videos based on resolution, filetype and codec
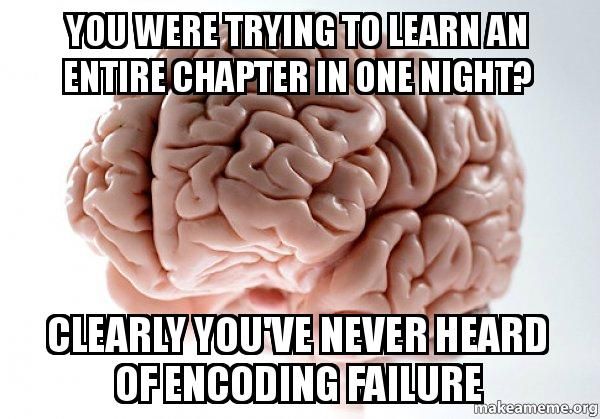
Its been quite handy recently to re-encode some old videos to x265/HEVC to save a bit (actually a fair bit :o) of disk space now that most devices at home can playback x265 videos in hardware. The main downside is its realtivly slow and finding the videos I wanted was a bit of a pain in the ass. Encoding lends itself to a nice little batch script though so here is a small (hacky) bash script that utilises mediainfo and handbrake cli to find the videos in a directoy and process them.
#!/bin/bash
# Notes:
# This basic script looks to match videos based on the codec type and also the resolution
# of the video then process them with HandbrakeCli. Options below to configure the match
# params for the script including the resolution, filetype and codec. The script will then
# process all matches and move the original files to a different folder so they are easy
# to remove once you are happy with the results. Output options include the filetype but
# also the encoding parameters for HandBrakeCli. Requires handbrakecli and mediainfo to
# both be installed on the system.
#
# For Ubuntu: sudo apt install mediainfo handbrake-cli
#
# Options:
# First off only process videos with a width of pixels great than this variable
RES=2000
# Only process these filetypes
INTYPE="avi"
# Only process videos with the video codec type as below
CODEC="MJPG"
# Following encoding move the original file to here. Do not forget the trailing /
POST="../originals/"
# Filetype for video output
OUTTYPE="mkv"
# Encoding params for HandBrakeCLI
PARAMS="-e x265 -q 20 -E av_aac"
########################################################################################
mkdir -p $POST
for fname in *.$INTYPE ; do
finfo=(`mediainfo --Output="Video;%Width% %CodecID%" $fname`)
if [ -z "${finfo[0]}" ] ; then
echo " --- $fname has unset resolution of $fsize so SKIPPING .... "
continue
elif [ -z "${finfo[1]}" ] ; then
echo " --- could not read the codec info for $fname so SKIPPING .... "
continue
elif [ "${finfo[1]}" != "$CODEC" ] ; then
echo " --- $fname has codec of ${finfo[1]} so SKIPPING .... "
continue
else
if [ ${finfo[0]} -gt $RES ] ; then
echo " +++ $fname has resolution of $fsize which is greater than $RES so PROCESSING "
filename=$(basename $fname)
filename=${filename%.*}
HandBrakeCLI -i $fname -o $filename.$OUTTYPE $PARAMS
echo " +++ moving original ..."
mv $fname $POST
echo " +++ moved to $POST"
echo -e "\n\n"
else
echo " --- $fname has resolution of ${finfo[0]} which is less than $RES so SKIPPING .... "
fi
fi
done
echo -e "\n All done :) Script took $SECONDS seconds to complete \n"
m00nie